
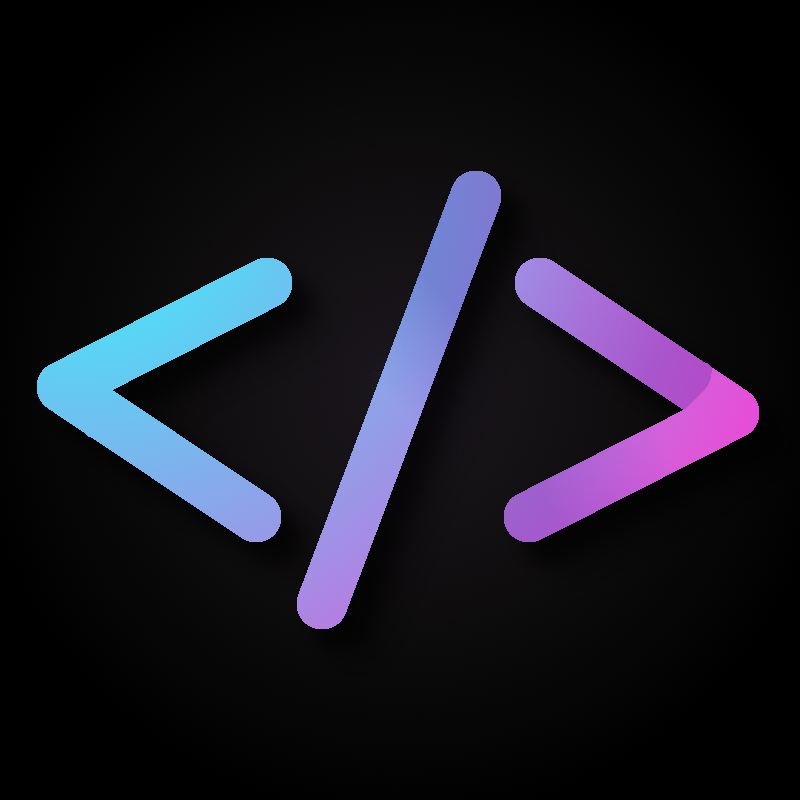
Based on some places I used to work, upper management seemed convinced that the “idea” stage was the hardest and most important part of any project, and that the easy part is planning, gathering requirements, building, testing, changing, and maintaining custom business applications for needlessly complex and ever changing requirements.
Having used PHP and Java extensively in my career, it’s always entertaining to read what people think about these languages.